Docker and Docker Compose are powerful tools for developers, providing an efficient way to develop, test, and deploy applications in a consistent environment. Here’s why you should consider using Docker and Docker Compose, along with code examples to illustrate their use.
Benefits of Docker
1. Consistency Across Environments: Docker ensures that your application runs the same way in development, testing, and production environments by encapsulating it and its dependencies in containers.
2. Isolation: Docker containers provide isolated environments, preventing conflicts between applications running on the same system.
3. Scalability: Docker makes it easy to scale applications horizontally by running multiple instances of containers.
4. Efficiency: Docker containers are lightweight and use fewer resources compared to virtual machines.
Docker Workflow
Creation of Dockerfile:
The developer initiates the process by creating a Dockerfile. This file outlines a set of instructions for building the Docker image, specifying the base image, application files, dependencies, and environment setup.
Building the Docker Image:
Next, the developer uses the Dockerfile to build a Docker image. This image encapsulates the application along with its runtime environment and dependencies.The command used for this step is:
docker build -t <image_name>:<tag> .
Shell
Pushing the Docker Image to a Registry:
After building the image, the developer pushes it to a Docker registry. This action makes the image available for
others to pull and use. The command for pushing the image is:
docker push <registry>/<image_name>:<tag>
Shell
By pushing the image to the registry, it becomes accessible for deployment on various servers.
Pulling the Docker Image:
On a remote server, the developer pulls the Docker image from the registry using the docker-compose pull command.
The command used is:
docker-compose pull <image_name>
Shell
Starting the Application:
After pulling the image, the developer starts the application with the docker-compose up command. This step creates and starts the Docker container from the pulled image.
The command executed is:
docker-compose up -d
Shell
The -d flag indicates that the container runs in detached mode, allowing the developer to use the terminal for other tasks while the container runs in the background.
Running Docker Containers on Any Docker-Enabled Machine:
Finally, the observer notes that the developer can run the Docker container on any machine equipped with Docker. This guarantees a consistent environment across different platforms, enhancing the application's portability and manageability.
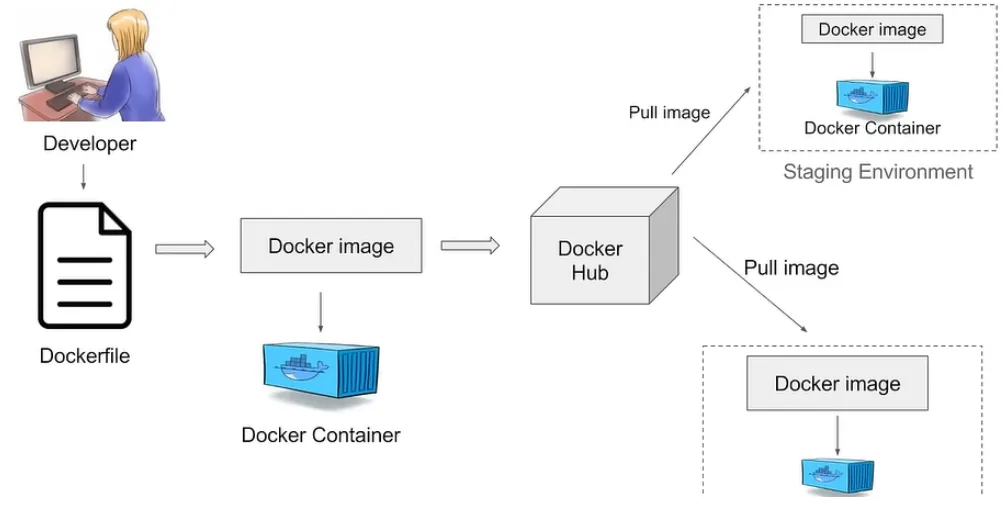
From Dockerfile to running docker container
Benefits of Docker Compose
1. Simplified Multi-Container Applications: Docker Compose allows you to define and manage multi-container Docker applications using a single YAML file.
2. Ease of Use: With a single command, you can start, stop, and rebuild all the services defined in a `docker-compose.yml` file.
3. Networking: Docker Compose sets up a network for your application’s services, enabling them to communicate with each other.
4. Volume Management: It allows for easy management of data volumes, ensuring persistent data across container restarts.
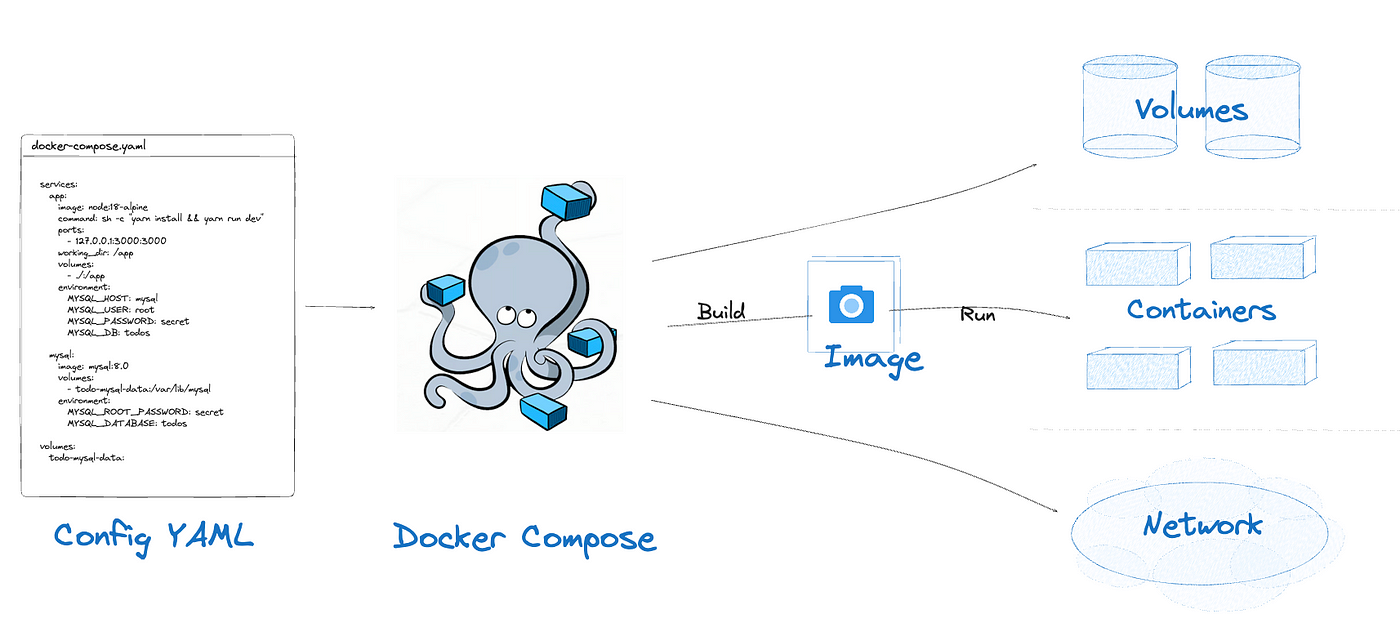
Docker compose
Code Examples
Example 1: Dockerfile
Create a Dockerfile to define the environment for a simple Python application.
Docker
Docker
# Use the official Python image from the Docker Hub
FROM python:3.9-slim
# Set the working directory inside the container
WORKDIR /app
# Copy the requirements.txt file and install dependencies
COPY requirements.txt .
RUN pip install --no-cache-dir -r requirements.txt
# Copy the rest of the application code
COPY . .
# Command to run the application
CMD ["python", "app.py"]
YAML
Example 2: docker-compose.yml
Create a `docker-compose.yml` file to define and manage a multi-container application.
version: '3.8'
services:
web:
build: .
ports:
- "5000:5000"
volumes:
- .:/app
environment:
- FLASK_ENV=development
depends_on:
- db
db:
image: postgres:13
environment:
POSTGRES_USER: myuser
POSTGRES_PASSWORD: mypassword
POSTGRES_DB: mydatabase
volumes:
- pgdata:/var/lib/postgresql/data
volumes:
pgdata:
Example 3: Running Docker and Docker Compose
1. Build and Run with Docker
# Build the Docker image
docker build -t my-python-app .
# Run the Docker container
docker run -p 5000:5000 my-python-app
2. Using Docker Compose
# Start the application
docker-compose up
# Stop the application
docker-compose down
# Rebuild the application
docker-compose up --build
Docker and Docker Compose streamline the process of developing, testing, and deploying applications by providing consistent and isolated environments. Docker allows you to package your application with all its dependencies, ensuring it runs smoothly across different environments. Docker Compose simplifies the management of multi-container applications, making it easier to define, start, and stop services with a single configuration file. By adopting Docker and Docker Compose, you can improve the efficiency, scalability, and reliability of your application development workflow.